Javascript Objects
I've been trying to learn some JavaScript (again). I've got a couple of books which are both pretty useful JavaScript: The Good Parts and The Art & Science of JavaScript. I would also strongly recommend reading JavaScript, 5 ways to call a function; an excellent blog post.
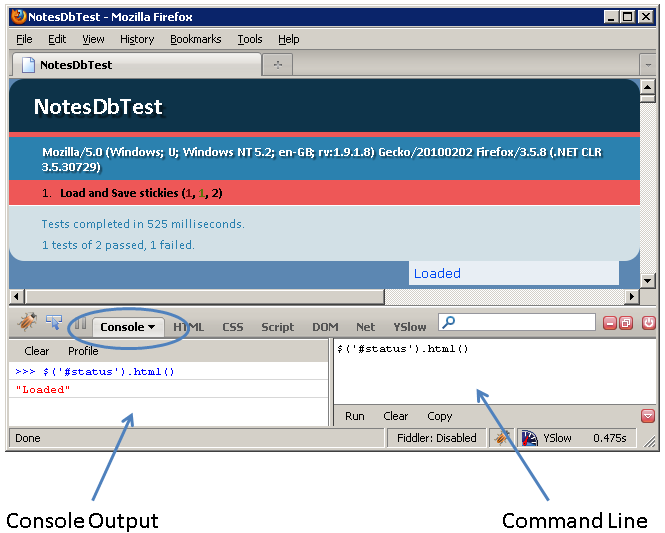
Command Line
Firebug has a JavaScript command line, which is useful for trying things out. For it to work correctly you need to be pointing at a real web page. To reset the state of the script, you simply refresh the web page.
The same tab also has a console which you can log too. It can do a bunch of stuff, but the most useful commands are:
- console.log('text') which just writes to the console.
- console.dir(object) which lists the objects properties.
Objects
JavaScript primitive types are bool, string and number. Everything else, including function, is an Object. An object is effectively a hash. The hash can have keys (properties) of any type, though they are normally strings. The values can be any type, including other objects and functions.
Object literals are a convenient way to make new objects:
//Empty object
var myPaper = {};
//Square bracket notation can get\set keys of any type
myPaper['newspaper'] = 'The Telegraph';
//The dot notation can get\set string keys (aka expando properties)
myPaper.cost = 1.2;
//A function can be assigned to a property
myPaper.gravitas = function(){
console.log("I have it");
};
//The for-in loop is used to examine properties on an object
for(key in myPaper){
console.log(key+':'+myPaper[key]);
}
An object literal can also define the properties in-line:
//Can define the properties within the object literal
var yourPaper = {
newspaper: "The Sun",
cost: 0.3,
gravitas: function(){
console.log("You dont");
}
};
//Can examine the object with console.dir
console.dir(yourPaper);
Functions
Functions are a type of object. They can be assigned to properties, variables and passed to other functions. When a function is created, it is given a prototype property which is itself an object.
//named function
function add(a, b) {
return a+b;
}
console.log(add(3,5));
console.dir(add);
// => 8
// => prototype Object{}
// anonymous function assigned to variable
var myFunc = function(a, b) {
return a+b;
}
console.log(myFunc(7,11));
console.dir(myFunc);
// => 18
// => prototype Object{}
New
Another way to make objects is with the new operator. New is called on a function. When a function is called this way its behaviour is changed:
- A new object is created.
- The new object has a hidden link to the original functions prototype object.
- "this" is bound to the new object.
- The return value from the function is ignored and the new object is returned instead.
function Foo() { };
Foo.prototype = {
write: function() {
console.log('woop');
},
writeAll: function() {
this.write();
}
};
var myFoo = new Foo();
myFoo.writeAll();
//=> woop
And that's the basic mechanics of creating objects in JavaScript.